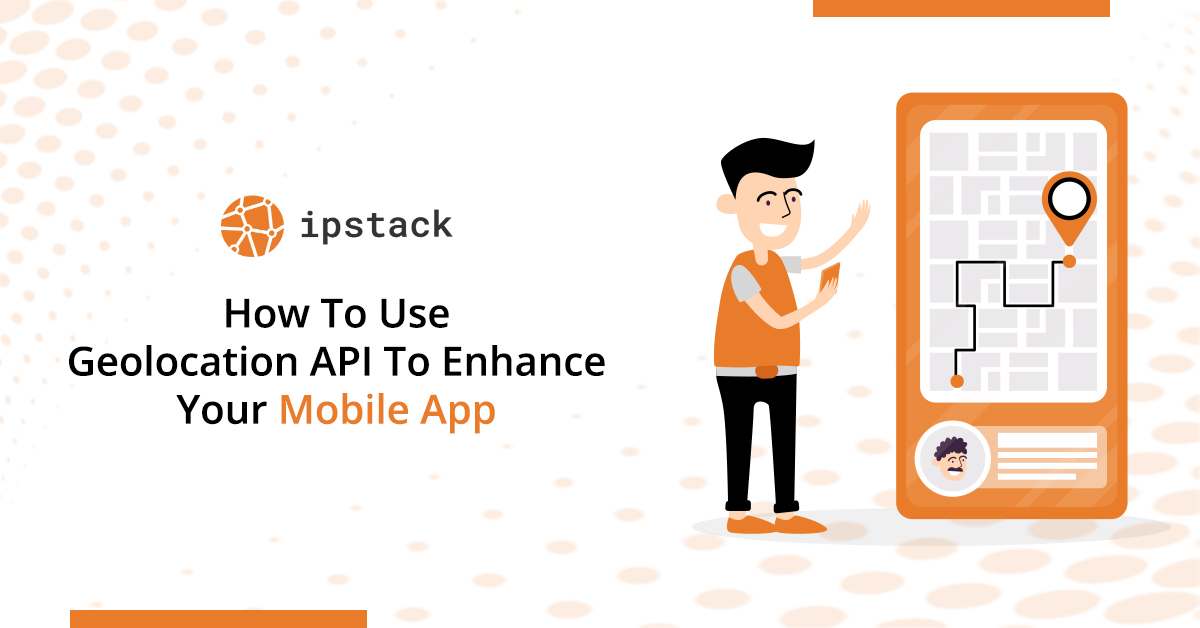
How To Use Geolocation API To Enhance Your Mobile App
In this roundup, you will learn how to use a geolocation API to enhance your mobile app. We will provide some JavaScript examples you can study and share with your developers.
In this how-to, we will introduce you to ipstack, a global IP database service that provides accurate location data and location information. ipstack offers a geolocation object that can be used to determine a user's location, position, and current location. It also provides geolocation APIs that allow developers to access position data and geographic location. ipstack is known for providing the most accurate location data, even for mobile devices. With ipstack, developers can easily access geo IP APIs to get the most up-to-date information about a user's location.
Best IP Geolocation API
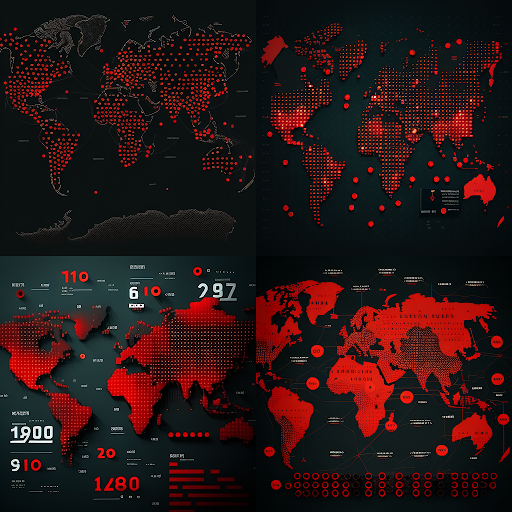
An IP geolocation API is a web API that allows developers to access IP geolocation data in various formats. It is an essential tool for developers who want to add location-aware features to their mobile apps. IP geolocation API enables developers to easily identify the location of a user's device, which can be used to improve the user experience and add targeted content.
ipstack is one of the best IP geolocation APIs available on the market. It offers a simple, easy-to-use API that allows developers to integrate IP geolocation into their mobile apps. ipstack provides accurate location data for over two billion IP addresses worldwide. It also provides detailed geolocation data such as city, region, country, zip code, time zone, and coordinates.
Why Use ipstack to Enhance Your Mobile App?
ipstack has code examples for its documentation in both PHP and JavaScript. Even though ipstack's geolocation API isn't tied to any platform or language, we can use it with Swift, Objective-C, Java, and Kotlin. This means developers can use ipstack's geolocation API to build mobile apps for any platform. This roundup will use JavaScript examples to show how to add ipstack to your mobile app.
JavaScript is often used when making mobile apps, but usually in hybrid or cross-platform development frameworks like React Native, Ionic, or Cordova. These frameworks allow developers to write mobile apps in JavaScript that can run on multiple platforms (e.g., iOS and Android).
Are IP Addresses Assigned to Mobile Devices More Geographically Accurate Than IP Addresses Assigned to Computers?
The accuracy of geolocation data based on IP addresses can vary depending on several factors, including the type of device and the network used to connect to the internet.
In general, IP addresses assigned to mobile devices are often more geographically accurate than those assigned to computers. This is because mobile devices typically connect to the internet using cellular networks, which have more specific geographic areas and a higher density of cell towers. As a result, the IP addresses assigned to mobile devices can be mapped to a more precise location compared to those assigned to computers.
However, the accuracy of geolocation data based on IP addresses can still vary significantly depending on various factors, such as the type of network, the location of the ISP's server, and the presence of VPNs or proxies that can obscure the device's true location. Therefore, while IP addresses assigned to mobile devices may be more accurate than those assigned to computers, it is still important to consider the limitations of IP-based geolocation data when using it for location-based services or other applications.
Using Your User's Position to Create Personalized Services
ipstack can enhance your mobile app by providing geolocation information that can be used to create personalized and location-based features for your app's users. Here are some examples of how you could use the geolocation data provided by Ipstack to enhance your mobile app:
- Personalized content: You can use the user's location data to display personalized content, such as local news, weather, or events.
- Location-based services: You can use the user's location data to provide location-based services, such as local restaurant recommendations or nearby parking options.
- Targeted advertising: You can use the user's location data to provide targeted advertising based on their location, such as promoting local businesses or events.
- Geofencing: You can use the user's location data to create geofences, which are virtual boundaries that trigger specific actions when the user enters or exits a particular location. For example, you could use geofencing to notify the user when they enter a specific store or venue.
Let's review how the above ideas can be implemented using ipstack and JavaScript code. Note that these examples are just a starting point, and you will need to adapt them to your specific use case and mobile app development framework. Additionally, it's important to make sure that you follow the ipstack API documentation and terms of service when using the API in your mobile app.
- Personalized content:
This code retrieves the user's IP address by making an API request to ipify.org. Then, it makes another API request to ipstack API using the retrieved IP address to get the user's geographical location data, such as city and region. Finally, it displays personalized content based on the user's location by setting the content of an HTML element with the ID "content" to a string that includes the user's city and region.
// Get the user's IP address using an API like ipify.org
fetch('https://api.ipify.org?format=json')
.then(response => response.json())
.then(data => {
const ipAddress = data.ip;
const ipstackApiKey = 'YOUR_IPSTACK_API_KEY_HERE';
const url = `http://api.ipstack.com/${ipAddress}?access_key=${ipstackApiKey}`;
// Make a request to the ipstack API to get the user's geolocation data
fetch(url)
.then(response => response.json())
.then(data => {
const userCity = data.city;
const userRegion = data.region_name;
// Display personalized content based on the user's location
document.getElementById('content').innerHTML = `Welcome to ${userCity}, ${userRegion}!`;
});
});
This code retrieves the user's IP address by making an API request to ipify.org. Then, it makes another API request to ipstack API using the retrieved IP address to get the user's geographical location data, such as latitude and longitude. Next, it uses the latitude and longitude to make a request to the Google Places API to find nearby restaurants. Finally, it displays the nearby restaurants to the user.
// Get the user's IP address using an API like ipify.org
fetch('https://api.ipify.org?format=json')
.then(response => response.json())
.then(data => {
const ipAddress = data.ip;
const ipstackApiKey = 'YOUR_IPSTACK_API_KEY_HERE';
const url = `http://api.ipstack.com/${ipAddress}?access_key=${ipstackApiKey}`;
// Make a request to the ipstack API to get the user's geolocation data
fetch(url)
.then(response => response.json())
.then(data => {
const userLatitude = data.latitude;
const userLongitude = data.longitude;
// Use the user's location data to provide location-based services
// For example, find nearby restaurants using the Google Places API
const placesApiKey = 'YOUR_GOOGLE_PLACES_API_KEY_HERE';
const placesUrl = `https://maps.googleapis.com/maps/api/place/nearbysearch/json?location=${userLatitude},${userLongitude}&radius=500&type=restaurant&key=${placesApiKey}`;
fetch(placesUrl)
.then(response => response.json())
.then(data => {
const nearbyRestaurants = data.results;
// Display nearby restaurants to the user
});
});
});
- Targeted advertising:
This code uses an API like ipify.org to get the user's IP address. It then uses this IP address to make a request to the ipstack API to get the user's geolocation data, including their country. The code then uses the user's country data to provide targeted advertising based on location. For example, if the user is in the United States, it may display a local business promotion that is relevant to users in the US. If the user is in Canada, it may display a local business promotion that is relevant to users in Canada. Note that the code does not actually display any specific business promotion, as this functionality is not included in the provided code. Instead, it provides a general framework for using geolocation data to provide targeted advertising.
// Get the user's IP address using an API like ipify.org
fetch('https://api.ipify.org?format=json')
.then(response => response.json())
.then(data => {
const ipAddress = data.ip;
const ipstackApiKey = 'YOUR_IPSTACK_API_KEY_HERE';
const url = `http://api.ipstack.com/${ipAddress}?access_key=${ipstackApiKey}`;
// Make a request to the ipstack API to get the user's geolocation data
fetch(url)
.then(response => response.json())
.then(data => {
const userCountry = data.country_name;
// Use the user's location data to provide targeted advertising
// For example, promote local businesses to users in specific countries
if (userCountry === 'United States') {
// Display a local business promotion to users in the US
} else if (userCountry === 'Canada') {
// Display a local business promotion to users in Canada
}
});
});
- Geofencing:
In this example, you will learn how to use geolocation services to create geofencing. The code below uses the user's current location to check if they are inside a geofence around New York City. If the user is inside the geofence, a welcome message is displayed. A message indicating they are not in New York City is displayed if the user is outside the geofence. The getDistanceFromLatLonInM function is used to calculate the distance between two sets of coordinates, which is then compared to the radius of the geofence to determine if the user is inside or outside the fence.
// Get the user's current location using the Geolocation API
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(position => {
const userLatitude = position.coords.latitude;
const userLongitude = position.coords.longitude;
// Set up a geofence around a specific location
const fenceLatitude = 40.7128; // New York City latitude
const fenceLongitude = -74.0060; // New York City longitude
const fenceRadius = 500; // Fence radius in meters
const distance = getDistanceFromLatLonInM(userLatitude, userLongitude, fenceLatitude, fenceLongitude);
// Check if the user is inside the geofence
if (distance <= fenceRadius) {
// User is inside the geofence, trigger a specific action
alert('Welcome to New York City!');
} else {
// User is outside the geofence
alert('You are not in New York City.');
}
});
} else {
alert('Geolocation is not supported by your device.');
}
// Helper function to calculate the distance between two coordinates
function getDistanceFromLatLonInM(lat1, lon1, lat2, lon2) {
const earthRadius = 6371000; // Earth's radius in meters
const dLat = deg2rad(lat2 - lat1);
const dLon = deg2rad(lon2 - lon1);
const a =
Math.sin(dLat / 2) * Math.sin(dLat / 2) +
Math.cos(deg2rad(lat1)) * Math.cos(deg2rad(lat2)) * Math.sin(dLon / 2) * Math.sin(dLon / 2);
const c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a));
const distance = earthRadius * c;
return distance;
}
// Helper function to convert degrees to radians
function deg2rad(deg) {
return deg * (Math.PI / 180);
}
Using Global IP Database Services in Your Mobile Client's Device Location Services
In this round-up you have learned how to enhance your mobile app's user experience by using a geolocation web API like ipstack.
The ipstack API can provide accurate location information on your users, allowing you to create personalized content, location-based services, targeted advertising, and geofencing.
You have also seen code examples in JavaScript, a language commonly used in hybrid and cross-platform mobile app development frameworks like React Native, Ionic, and Cordova.
Finally, you now know that while IP addresses assigned to mobile devices are generally more geographically accurate than those assigned to computers, the accuracy of geolocation data based on IP addresses can still vary depending on various factors.