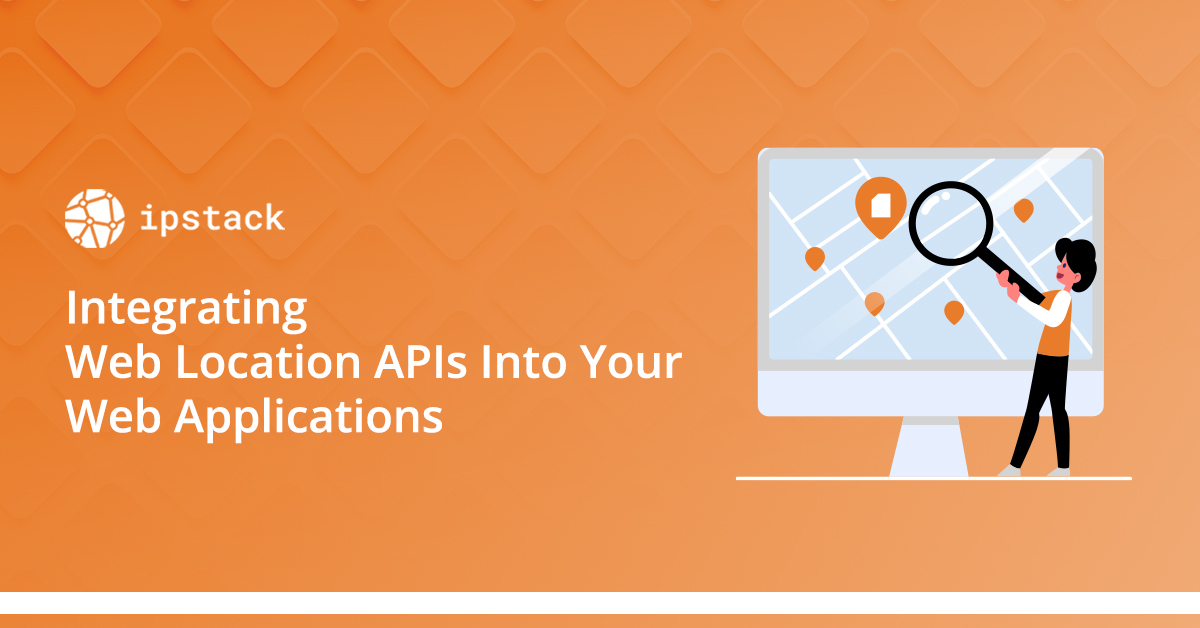
Integrating Web Location APIs Into Your Web Applications
Are you looking to use accurate location data about user location to serve your web visitors with personalized services?
In this roundup, we will help you to become familiar with Web Location APIs (also known as Geolocation API), and we will introduce you to IPstack and show you how you can make a simple web application that gets the geolocation longitude and latitude position data of a website visitor's last IP address.
We will also explain how Web location APIs gather information and whether you can expect accurate results. It is worth noting, at the start, that the actual physical location of a user's position is often different from the precise physical location information of their last recorded IP address, especially as their position changes.
Locations of a website user's last known IP addresses can provide a great start to identifying who your returning visitors are, their country, and the nearest major city. With this information, you can collate user activity to timestamp information about when your website visitors log in and begin building more detailed information about them.
What is Geolocation API?
Integrating geolocation APIs into your web applications can provide a range of benefits, including:
- Personalization: Location-based services allow you to personalize your web application for each user based on their location, which can improve the user experience and increase engagement.
- Navigation: Using geolocation APIs, you can provide your users with accurate and reliable directions to a destination, which can be particularly useful for applications focused on transportation or tourism.
- Marketing: geolocation APIs can enable targeted marketing campaigns based on a user's location, increasing the effectiveness of your advertising and promotional efforts.
- Analytics: By collecting location data, you can gain insights into how users interact with your web application, which can help you optimize your design and content.
The difficulty of integrating geolocation APIs into your web application depends on various factors, including the specific API you are using, your level of expertise in web development, and the complexity of your application. Most APIs provide clear documentation and code samples, making integration relatively straightforward for experienced developers. However, if you're new to web development or working with a particularly complex application, it may require more time and effort to integrate location-based services effectively. Pick a geolocation API service with very clear documentation to help you start. We recommend IPstack for its clear user documentation and FREE API key to get started.
Locate the User's Position
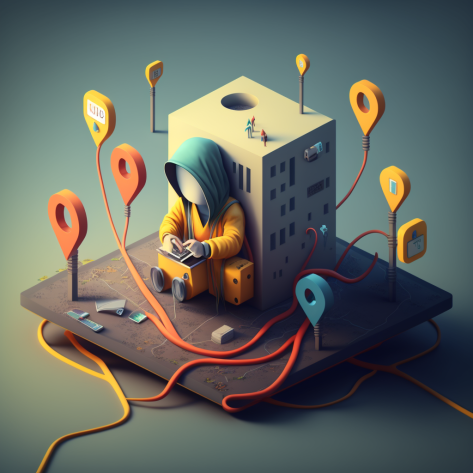
If you're wondering how geolocation APIs locate your position, they typically use various methods such as GPS, Wi-Fi triangulation, cellular triangulation, IP address geolocation, and HTML5 geolocation. The specific method depends on your device's hardware and software features and the algorithms programmed into the API. For example, if your device has a GPS sensor, the API may use that to determine your location. Alternatively, if your device doesn't have a GPS sensor, the API may use Wi-Fi, cellular triangulation, or even your IP address. Once the API has determined your location, it can be used to provide you with location-based services, such as directions, recommendations, and weather updates.
Regional Internet Registries (RIR)
Web location APIs rely heavily on IP address data to determine a user's location. Regional Internet Registries (RIRs) are crucial in this regard because they manage and allocate IP addresses, which are critical components of location-based services. The accuracy and availability of location-based services depend on the reliability and consistency of IP address data.
Regional Internet Registries (RIRs) are relevant to web location APIs because they are responsible for managing and allocating IP addresses, which are a crucial component of location-based services.
RIRs ensure that IP addresses are allocated efficiently and effectively, which helps to maintain the accuracy and reliability of location-based services. By preventing IP addresses from being overused or misused, RIRs also play a critical role in maintaining the security and stability of the internet as a whole. This, in turn, ensures that users can access and use online services and content without interruption.
While users may not directly interact with RIRs, these organizations collaborate with other internet organizations to ensure the security and stability of the internet. As a result, RIRs play a vital role in ensuring that internet resources are used effectively and efficiently by their member organizations, including Internet Service Providers (ISPs) and other organizations that require internet resources.
RIRs are essential to the functioning of web location APIs by providing accurate and reliable IP address data. The allocation of IP addresses by RIRs helps to maintain the accuracy and reliability of location-based services while also ensuring the security and stability of the internet as a whole. RIRs are crucial in ensuring that users can access and use online services and content without interruption.
Finding Your User's Position, Which Geolocation API to Choose?
For the reasons explained above, we have demonstrated many different technical methods that different API service providers may utilize to ascertain a website visitor's location data. Depending on your web application use case, you have several choices for which API service to test and implement for retrieving the nearest known IP locations to your user. We recommend IPstack API to begin with as it offers very scalable plans to increase your API call allowance as your business grows. To help your web application find your user's position IPstack utilizes a global IP database service to provide you with IP geolocation.
Limitations of Geolocation API Returns
IPstack is a geolocation API that provides location data based on a user's IP address. While this method can be useful for determining a user's approximate location, it can also be inaccurate for many reasons:
- Use of VPNs or proxies: If a user is accessing your web application through a VPN or proxy, the IP address that IPstack detects may not accurately reflect the user's true location. Instead, the location of the VPN or proxy server will be detected, leading to inaccurate location data.
- Shared IP addresses: In some cases, multiple users may share the same IP address. This can occur when a user is accessing the internet through a public Wi-Fi or a corporate network. In these cases, ipstack may not be able to accurately determine the location of a particular user.
- Dynamic IP addresses: Some internet service providers (ISPs) use dynamic IP addresses, which means that the IP address assigned to a user can change over time. If IPstack is not updated with the correct IP address, it may provide location data based on a previous IP address, leading to inaccurate results.
- Incomplete or outdated databases: Geolocation APIs rely on databases of IP addresses and their associated locations. If databases are incomplete or outdated, it can lead to inaccurate location data.
- Interference from firewalls or security software: Some firewalls or security software can interfere with IPstack's ability to accurately determine a user's location, leading to inaccurate results.
It's important to note that IPstack is just one of many geolocation APIs available, and the accuracy of the location data provided by any API can vary depending on several factors. Developers should carefully consider their specific use case and the potential limitations of any geolocation API they use. Additionally, you may want to consider using multiple geolocation APIs or other location detection methods to improve the accuracy of your results.
Getting Started with IPstack
With a few lines of Python code and an IPstack API key, you are ready to begin finding out a user's current location or at least their leading IP.
Longitude and Latitude Example
The code below sends a request to the IPstack API with the access key, retrieves the JSON response, and extracts the latitude and longitude values from the JSON data. It then prints the latitude and longitude to the console. You can use these values for further processing or display them in a user interface. In the next code, we will show you how IPstack can work together with other APIs in a web application.
import requests
ipstack_api_key = "YOUR API KEY"
ipstack_url = f"http://api.ipstack.com/check?access_key={ipstack_api_key}"
response = requests.get(ipstack_url)
data = response.json()
latitude = data["latitude"]
longitude = data["longitude"]
print(f"Latitude: {latitude}, Longitude: {longitude}")
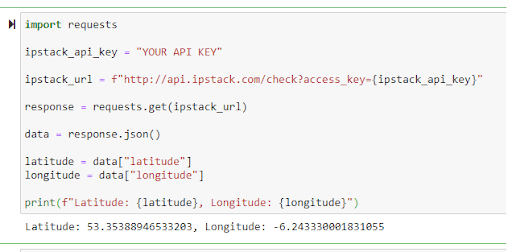
Preparing an Application That Uses Geolocation Location Information
The Python code below retrieves your IP address location using IPstack API, weather information for the nearest city of your IP location using weatherstack API, and news data for your IP address's city location using mediastack and then prints the results to your console. You must have Python installed on your computer and API keys for the IPstack, weatherstack, and mediastack.
The code first assigns the API keys to variables. Then, it uses the IPstack API to retrieve the user's city based on their IP address. It does this by making a GET request to the IPstack API URL, passing the API key as a parameter, and specifying that it only wants the city field in the response. It then parses the JSON response and extracts the city value.
Combining API Services Together
Next, the code uses the weatherstack API to retrieve the current temperature and weather description for the user's city. It does this by making a GET request to the weatherstack API URL, passing the API key and the city as parameters. It then parses the JSON response and extracts the temperature and weather description values.
Finally, the code uses the mediastack API to retrieve the 5 most recent news articles relevant to the user's city. It does this by making a GET request to the mediastack API URL, passing the API key, and the city as a keyword parameter, and specifying additional parameters such as language and sort order. It then parses the JSON response and extracts the article titles and URLs.
The retrieved data is then printed to your console.
To run the following code, you will need to obtain a free API key account from the following API Layer services (click on the links below to sign up):
Replace the API key variables with your own API keys for the IPstack, weatherstack, and mediastack APIs. Save the code to a Python file and run it from your command line or Python IDE.
import requests
ipstack_api_key = "Your API key"
mediastack_api_key = "Your API key"
weatherstack_api_key = "Your API key"
# Retrieve the location data using the ipstack API
ipstack_url = f"http://api.ipstack.com/check?access_key={ipstack_api_key}"
ipstack_params = {"fields": "city"}
ipstack_response = requests.get(ipstack_url, params=ipstack_params)
ipstack_data = ipstack_response.json()
city = ipstack_data["city"]
# Retrieve the weather data using the weatherstack API
weatherstack_url = f"http://api.weatherstack.com/current?access_key={weatherstack_api_key}&query={city}"
weatherstack_response = requests.get(weatherstack_url)
weatherstack_data = weatherstack_response.json()
temperature = weatherstack_data["current"]["temperature"]
weather_description = weatherstack_data["current"]["weather_descriptions"][0]
# Retrieve the news data using the mediastack API
mediastack_url = f"http://api.mediastack.com/v1/news?access_key={mediastack_api_key}&keywords={city}&languages=en&sort=published_desc&limit=5"
mediastack_response = requests.get(mediastack_url)
mediastack_data = mediastack_response.json()
articles = mediastack_data["data"]
# Print the retrieved data
print(f"Current temperature in {city} is {temperature}°C and {weather_description}.")
print(f"Here are the 5 most recent news articles relevant to {city}:")
for article in articles:
print(article["title"])
print(article["url"])
print()
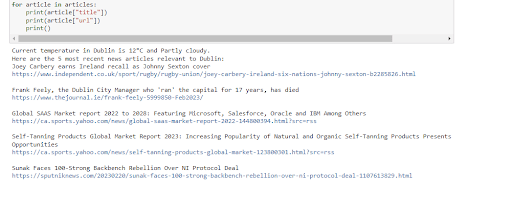
If you like how this code runs on your computer, you can adapt it slightly to make a Python Flask application or JavaScript and deploy it to the web.
Deploy Simple Flask Website Application Using IPstack
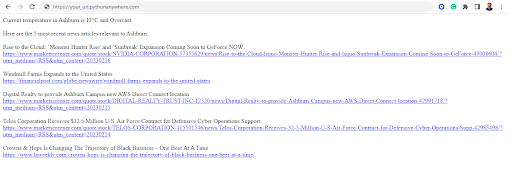
The following code is an adaption of the Python code above, which can be used with hosting providers that support Python Flask applications such as PythonAnywhere. When deployed as a web app, users should be able to see their IP address nearest city location in the web browser.
import requests
from flask import Flask, request
ipstack_api_key = "Your API key"
mediastack_api_key = "Your API key"
weatherstack_api_key = "Your API key"
app = Flask(__name__)
@app.route("/")
def get_info():
ipstack_url = f"http://api.ipstack.com/check?access_key={ipstack_api_key}"
ipstack_params = {"fields": "city"}
ipstack_response = requests.get(ipstack_url, params=ipstack_params)
ipstack_data = ipstack_response.json()
city = ipstack_data["city"]
weatherstack_url = f"http://api.weatherstack.com/current?access_key={weatherstack_api_key}&query={city}"
weatherstack_response = requests.get(weatherstack_url)
weatherstack_data = weatherstack_response.json()
temperature = weatherstack_data["current"]["temperature"]
weather_description = weatherstack_data["current"]["weather_descriptions"][0]
mediastack_url = f"http://api.mediastack.com/v1/news?access_key={mediastack_api_key}&keywords={city}&languages=en&sort=published_desc&limit=5"
mediastack_response = requests.get(mediastack_url)
mediastack_data = mediastack_response.json()
articles = mediastack_data["data"]
info = f"Current temperature in {city} is {temperature}°C and {weather_description}.
Here are the 5 most recent news articles relevant to {city}:"
for article in articles:
info += f"{article['title']}"
info += f"{article['url']}"
return info
if __name__ == "__main__":
app.run()
Note that if you are deploying this code to PythonAnywhere, a slight modification is required to the code to include request.headers (See their help guide 'How to get the IP addresses of clients for your web app'):
from flask import Flask, request
app = Flask(__name__)
@app.route("/")
def get_data():
ip_address = request.headers['X-Real-IP']
ipstack_api_key = "Your API key"
mediastack_api_key = "Your API key"
weatherstack_api_key = "Your API key"
# Retrieve the location data using the ipstack API
ipstack_url = f"http://api.ipstack.com/{ip_address}?access_key={ipstack_api_key}"
ipstack_params = {"fields": "city"}
ipstack_response = requests.get(ipstack_url, params=ipstack_params)
ipstack_data = ipstack_response.json()
city = ipstack_data["city"]
# Retrieve the weather data using the weatherstack API
weatherstack_url = f"http://api.weatherstack.com/current?access_key={weatherstack_api_key}&query={city}"
weatherstack_response = requests.get(weatherstack_url)
weatherstack_data = weatherstack_response.json()
temperature = weatherstack_data["current"]["temperature"]
weather_description = weatherstack_data["current"]["weather_descriptions"][0]
# Retrieve the news data using the mediastack API
mediastack_url = f"http://api.mediastack.com/v1/news?access_key={mediastack_api_key}&keywords={city}&languages=en&sort=published_desc&limit=5"
mediastack_response = requests.get(mediastack_url)
mediastack_data = mediastack_response.json()
articles = mediastack_data["data"]
# Create the response message
message = f"Current temperature in {city} is {temperature}°C and {weather_description}.
Here are the 5 most recent news articles relevant to {city}:"
for article in articles:
message += f"{article['title']}"
message += f"{article['url']}"
return message
if __name__ == '__main__':
app.run()
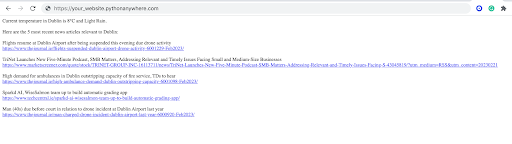
We hope you found this roundup useful and learned that not only is the API documentation for IPstack fairly easy to implement, but that when you integrate it with other APIs available from API Layer, you will soon be able to put together applications where different APIs communicate with each other to produce applications powered by geographical location data results with some simple scripting effort.