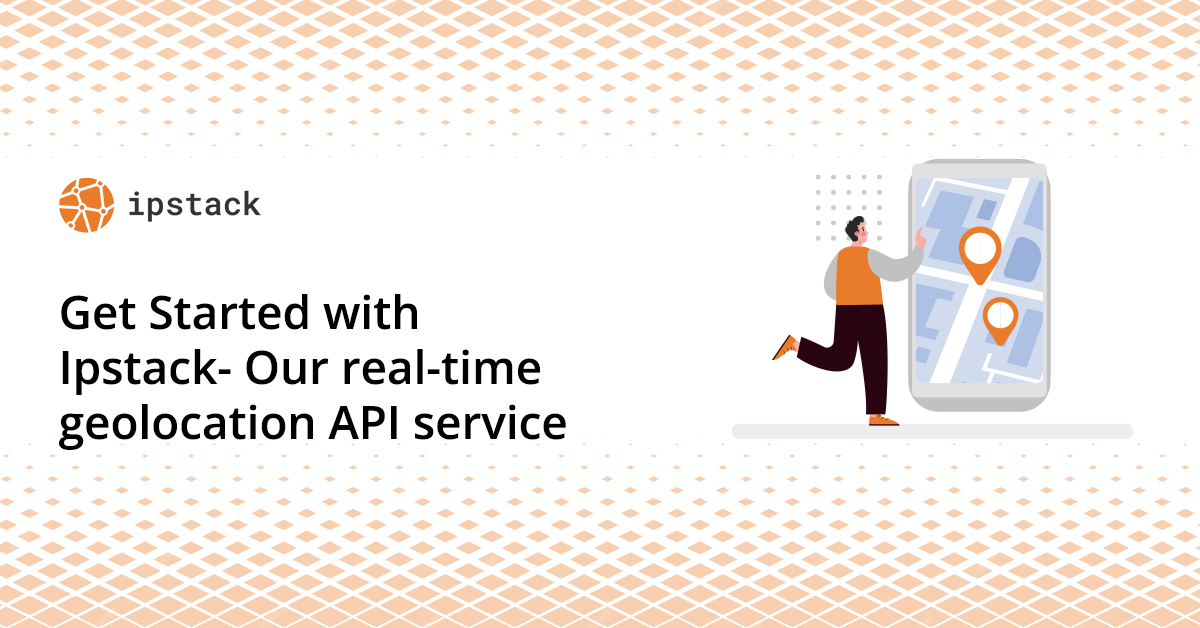
Get Started with IPstack- Our real-time geolocation API service
APIs that provide geolocation information corresponding to the IP address are called Geolocation APIs. Geolocation APIs are often preferred by businesses today.
After its popularity and popularity, the number of geolocation APIs has increased considerably. With the increasing number of geolocation APIs, it may seem difficult to find quality APIs. Today, IPstack is the most preferred geolocation APIthat provides geolocation information corresponding to the IP address.
In this article, we will talk about why the IPstack API is the most popular geolocation API and how to integrate it into the code side.
Why the IPstack API is most popular
The main reason why the IPstack API is popular is that it provides large data with an accuracy rate. The accuracy of geolocation information corresponding to IP addresses is of great importance for businesses. If businesses are working on statistics and analysis based on geolocations, the accuracy of geolocations helps businesses reach their goals easily.
Another reason is that the IPstack API provides very detailed geolocation information corresponding to an IP address. The IPstack API provides almost any location-related data businesses may need. For example, geolocation information corresponding to an IP address includes information such as the official currency of the location, currency symbol, timezone, continent name and continent code.
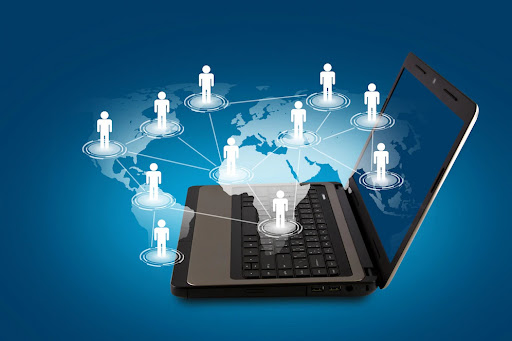
Ipstack API is loved by many developers for the flexibility it provides. For example, IPstack API can present the data corresponding to the IP address in both JSON and XML formats. It also has no affiliation with any programming language. For this reason, it can be easily integrated into many programming languages.
Detailed geolocation API information provided by IPstack API is obtained from very large global location databases. Thanks to the technological infrastructure of IPstack, detailed location information is obtained from large-scale databases by querying only in milliseconds and is transmitted to the user without any delay.
Today, IPstack API, which is used by many large-scale companies such as Microsoft, Samsung and HubSpot, provides technical support services to its users. It allows you to get instant technical support without any problems with integration or data.
In addition, the IPstack API has been developed to allow querying multiple location information at the same time. It can provide detailed geolocation information of more than one IP address in a list with a single query.
Integration IPstack API to Go programming language
To use the IPstack API, we need to obtain an API key. In order to obtain the API key, we must choose one of the flexible and affordable packages offered by the IPstack API and register.
After obtaining the API key, let's create a Go project and paste the following codes into it.
package main import ( "fmt" "net/http" "io/ioutil" ) func main() { url := "http://api.ipstack.com/134.201.250.155?access_key=c1*****51" method := "GET" client := &http.Client { } req, err := http.NewRequest(method, url, nil) if err != nil { fmt.Println(err) return } res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
When we run this code, the following IPstack API response will be printed on the console of the application.
{ "ip": "134.201.250.155", "type": "ipv4", "continent_code": "NA", "continent_name": "North America", "country_code": "US", "country_name": "United States", "region_code": "CA", "region_name": "California", "city": "Los Angeles", "zip": "90012", "latitude": 34.0655517578125, "longitude": -118.24053955078125, "location": { "geoname_id": 5368361, "capital": "Washington D.C.", "languages": [ { "code": "en", "name": "English", "native": "English" } ], "country_flag": "https://assets.ipstack.com/flags/us.svg", "country_flag_emoji": "\ud83c\uddfa\ud83c\uddf8", "country_flag_emoji_unicode": "U+1F1FA U+1F1F8", "calling_code": "1", "is_eu": false }, "time_zone": { "id": "America/Los_Angeles", "current_time": "2022-10-19T08:06:08-07:00", "gmt_offset": -25200, "code": "PDT", "is_daylight_saving": true }, "currency": { "code": "USD", "name": "US Dollar", "plural": "US dollars", "symbol": "$", "symbol_native": "$" }, "connection": { "asn": 25876, "isp": "Los Angeles Department of Water & Power" }, "security": { "is_proxy": false, "proxy_type": null, "is_crawler": false, "crawler_name": null, "crawler_type": null, "is_tor": false, "threat_level": "low", "threat_types": null } }
Now let's look at how we can get detailed geolocation information of more than one IP address with a single query. We will paste the code below into our Go project.
package main import ( "fmt" "net/http" "io/ioutil" ) func main() { url := "http://api.ipstack.com/134.201.250.155,72.229.28.185,110.174.165.78?access_key=c1*****51" method := "GET" client := &http.Client { } req, err := http.NewRequest(method, url, nil) if err != nil { fmt.Println(err) return } res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
When this code runs, the following information will be printed on the console of the application.
[ { "ip": "134.201.250.155", "type": "ipv4", "continent_code": "NA", "continent_name": "North America", "country_code": "US", "country_name": "United States", "region_code": "CA", "region_name": "California", "city": "Los Angeles", "zip": "90013", "latitude": 34.0453, "longitude": -118.2413, "location": { ... }, "time_zone": { ... }, "currency": { ... }, "connection": { ... }, }, { "ip": "72.229.28.185", "type": "ipv4", "continent_code": "NA", "continent_name": "North America", "country_code": "US", "country_name": "United States", "region_code":"NY", "region_name": "New York", "city": "New York", "zip": "10036", "latitude": 40.7605, "longitude": -73.9933, "location": { ... }, "time_zone": { ... }, currency": { ... }, "connection": { ... }, }, { "ip": "110.174.165.78", "type": "ipv4", "continent_code": "OC", "continent_name": "Oceania", country_code": "AU", "country_name": "Australia", "region_code": "NSW", "region_name": "New South Wales", "city": "Coffs Harbour", "zip": "2450", "latitude": -30.2963, "longitude": 153.1135, "location": { ... }, "time_zone": { ... }, "currency": { ... }, "connection": { ... }, } ]
Conclusion
There are many more reasons why the IPstack API is the most preferred geolocation API today. If you want to instantly obtain location information of your users and grow your business, take a look at the documentation prepared by IPstack API.