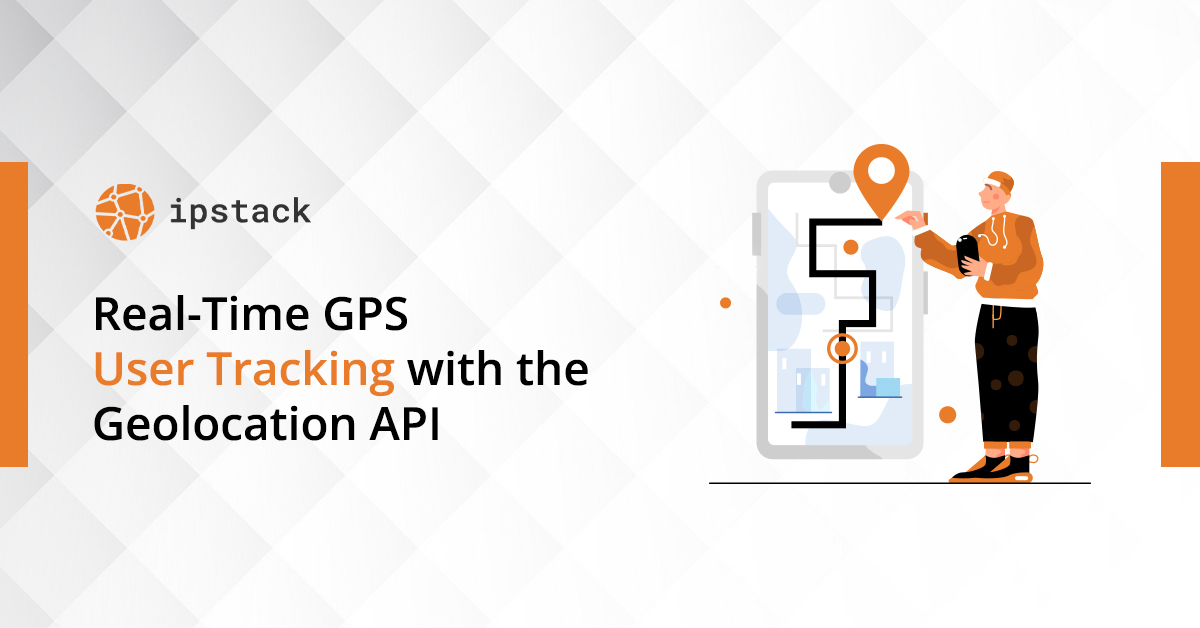
Real-Time GPS User Tracking with the Geolocation API
Today, the most popular applications generally work in real time. Such as messaging, e-commerce, and map applications. There are many ways to show the instant location of the user, especially in map applications. The most popular of these is to obtain the location of the user's IP address and make the user do many operations with the location data.
The location information corresponding to the IP address is provided by geolocation APIs in real time. The most popular web service providing geolocation is IPstack. With the API it provides, IPstack finds the location information corresponding to the IP address within milliseconds and provides it to the customers. Its very fast operation also supports real time operation. In addition, the IPstack API provides the location information of more than one IP address at the same time through a single endpoint.
It is the use of IPstack API, which is the most popular method of obtaining geolocation information of users in real time, by integrating it into applications and websites.,
Let's take a look at how we can integrate IPstack API, which provides real time geolocation service, into a python application.
Integration IPstack API with Python
Before we integrate the IPstack API into the application, we first need an API key to use the API. From here, we can choose one of the most affordable and flexible packages and obtain an API key.
Let's create a Python file on the desktop. For example 'main.py'. Let's paste the following codes into this Python file.
import http.client conn = http.client.HTTPSConnection("api.ipstack.com") payload = '' headers = {} conn.request("GET", "/134.201.250.155?access_key=c1*****51", payload, headers) res = conn.getresponse() data = res.read() print(data.decode("utf-8"))
The application is expected to obtain the detailed location information corresponding to the IP address 134.201.250.155 from IPstack and write it to the application console.
Let's run our python code by typing the following command from the command line.
python main.py
When this code runs, we see the following information in the application console.
{ "ip": "134.201.250.155", "type": "ipv4", "continent_code": "NA", "continent_name": "North America", "country_code": "US", "country_name": "United States", "region_code": "CA", "region_name": "California", "city": "Los Angeles", "zip": "90012", "latitude": 34.0655517578125, "longitude": -118.24053955078125, "location": { "geoname_id": 5368361, "capital": "Washington D.C.", "languages": [ { "code": "en", "name": "English", "native": "English" } ], "country_flag": "https://assets.ipstack.com/flags/us.svg", "country_flag_emoji": "\ud83c\uddfa\ud83c\uddf8", "country_flag_emoji_unicode": "U+1F1FA U+1F1F8", "calling_code": "1", "is_eu": false }, "time_zone": { "id": "America/Los_Angeles", "current_time": "2022-09-06T06:41:58-07:00", "gmt_offset": -25200, "code": "PDT", "is_daylight_saving": true }, "currency": { "code": "USD", "name": "US Dollar", "plural": "US dollars", "symbol": "$", "symbol_native": "$" }, "connection": { "asn": 25876, "isp": "Los Angeles Department of Water & Power" }, "security": { "is_proxy": false, "proxy_type": null, "is_crawler": false, "crawler_name": null, "crawler_type": null, "is_tor": false, "threat_level": "low", "threat_types": null } }
IPstack, which provides real time geolocation service, conveyed very detailed information about the location corresponding to the IP address.
Now let's take a look at how we can send requests to multiple IP addresses at the same time using the flexibility of the IPstack API and how we can make the incoming data in XML format.
Let's replace the code in 'main.py' with the following and run it again
import http.client
import http.client conn = http.client.HTTPSConnection("api.ipstack.com") payload = '' header = {} conn.request("GET", "/134.201.250.155,72.229.28.185,110.174.165.78?access_key=c1*****51&output=xml", payload, headers) res = conn.getresponse() data = res.read() print(data.decode("utf-8"))
After running this code, the following information is displayed in the application console.
<batch> <resultindex="0"> <ip>134.201.250.155</ip> <type>ipv4</type> <continent_code>NA</continent_code> <continent_name>North America</continent_name> <country_code>US</country_code> <country_name>United States</country_name> <region_code>CA</region_code> <region_name>California</region_name> <city>Los Angeles</city> <zip>90013</zip> <latitude>34.0453</latitude> <longitude>-118.2413</longitude> <location> [...] </location> <time_zone> [...] </time_zone> <currency> [...] </currency> <connection> [...] </connection> </result> <result index="1"> <ip>72.229.28.185</ip> <type>ipv4</type> <continent_code>NA</continent_code> <continent_name>North America</continent_name> <country_code>US</country_code> <country_name>United States</country_name> <region_code>NY</region_code> <region_name>New York</region_name> <city>New York</city> <zip>10036</zip> <latitude>40.7605</latitude> <longitude>-73.9933</longitude> <location> [...]</location> <time_zone> [...]</time_zone> <currency> [...]</currency> <connection> [...]</connection> </result> <result index="2"> <ip>110.174.165.78</ip> <type>ipv4</type> <continent_code>OC</continent_code> <continent_name>Oceania</continent_name> <country_code>AU</country_code> <country_name>Australia</country_name> <region_code>NSW</region_code> <region_name>New South Wales</region_name> <city>Coffs Harbour</city> <zip>2450</zip> <latitude>-30.2963</latitude> <longitude>153.1135</longitude> <location> [...]</location> <time_zone> [...]</time_zone> <currency> [...]</currency> <connection> [...]</connection> </result> </batch>
Conclusion
Applications using real time geolocation APIs provide users with many customized opportunities and conveniences. If you are considering real time geolocation APIs that increase user density in your applications, Ipstack provides you with great convenience. You can explore here.