Leveraging IP Stack for Enhanced Technical Support: An Overview
In the digital age, understanding your audience is pivotal. Not just from a marketing perspective, but for operational and technical support as well. One of the tools businesses employ for this purpose is the IP stack. By providing metadata for IP addresses, the IP stack can furnish critical insights about users, allowing support teams to offer tailored solutions.
What is IP Stack?
IP stack is a service that provides metadata for any given IP address. This metadata can include information such as the geographic location (down to the city), the Internet Service Provider (ISP), timezone, and even currency. For businesses that operate globally, this information is invaluable.
Business Scenario
Imagine you run a global online streaming service. Users from around the world access your platform. Suddenly, you receive multiple complaints from users in a specific region experiencing streaming issues. How do you swiftly identify the problem and rectify it?
This is where IP stack comes into play.
Step 1: Retrieve IP Metadata
First, when the user logs a support ticket, you can immediately pull metadata for their IP address.
import requests
def get_ip_metadata(ip_address, api_key): url = f"http://api.ipstack.com/{ip_address}?access_key={api_key}" response = requests.get(url) return response.json()
# Sample usage api_key = 'YOUR_IPSTACK_API_KEY' ip_address = '8.8.8.8' # example IP address metadata = get_ip_metadata(ip_address, api_key) print(metadata)
The provided code snippet is a simple Python script that fetches metadata for a given IP address using the IP stack API. Let's break it down step by step:
1. Importing the required module:
import requests
The script starts by importing the requests module, which is a popular Python library for making HTTP requests.
2. Defining the get_ip_metadata function:
def get_ip_metadata(ip_address, api_key):
url = f"http://api.ipstack.com/{ip_address}?access_key={api_key}" response = requests.get(url) return response.json()
This function is designed to fetch metadata for a given IP address from the IP stack API.
- ip_address and api_key are the function's parameters. The ip_address is the IP for which you want to get metadata, and api_key is the authentication key required by IP stack API to access its services.
- The url variable is constructed using an f-string (formatted string literal) to insert the IP address and API key into the URL of the IP stack endpoint.
- The line response = requests.get(url) sends a GET request to the constructed URL and captures the returned response.
- return response.json() then parses the JSON data from the API's response and returns it.
3. Sample usage
api_key = 'YOUR_IPSTACK_API_KEY' ip_address = '8.8.8.8' # example IP address metadata = get_ip_metadata(ip_address, api_key) print(metadata)
Here, the code provides a sample usage of the get_ip_metadata function:
- api_key should be set to your actual IP stack API key.
- ip_address is set to the example IP address '8.8.8.8' (which is a public Google DNS server).
- The function get_ip_metadata is called with the example IP address and API key, and its result (the metadata for the IP address) is stored in the metadata variable.
- Finally, the script prints out the fetched metadata to the console.
To use this script with the IP stack service, you'd need to replace 'YOUR_IPSTACK_API_KEY' with your actual IP stack API key.
Step 2: Analyze the Data
Once you have the data, you can analyze and categorize the issues. If multiple users from the same region or ISP are facing problems, it might suggest regional outages or ISP-specific problems.
python
from collections import defaultdict
# Sample data
tickets = [ {'user': 'Alice', 'ip': '8.8.8.8'}, {'user': 'Bob', 'ip': '8.8.4.4'}, # ... more tickets ]
issues_by_region = defaultdict(list) issues_by_isp = defaultdict(list)
for ticket in tickets: metadata = get_ip_metadata(ticket['ip'], api_key) region = metadata['region_name'] isp = metadata['isp']
issues_by_region[region].append(ticket['user']) issues_by_isp[isp].append(ticket['user'])
print("Issues by Region:", issues_by_region) print("Issues by ISP:", issues_by_isp)
This code snippet is designed to categorize user-reported issues based on region and ISP
(Internet Service Provider) using the IP stack API. Let's break down the provided code:
1. Importing the required module:
from collections import defaultdict
This imports the defaultdict class from the collections module. A defaultdict works like a regular dictionary but provides a default value for a key if the key isn't already present in the dictionary.
2. Sample Data:
tickets = [ {'user': 'Alice', 'ip': '8.8.8.8'}, {'user': 'Bob', 'ip': '8.8.4.4'}, # ... more tickets ]
This defines a list of sample tickets where each ticket is represented as a dictionary. Each dictionary contains a user's name and their associated IP address.
Each dictionary contains a user's name and their associated IP address.
3. Initializing defaultdicts:
issues_by_region = defaultdict(list) issues_by_isp = defaultdict(list)
Two defaultdicts are initialized with lists as their default values. These dictionaries will be used to categorize tickets based on the region (issues_by_region) and ISP
(issues_by_isp).
4. Looping through tickets and categorizing them:
for ticket in tickets:
metadata = get_ip_metadata(ticket['ip'], api_key) region = metadata['region_name'] isp = metadata['isp']
issues_by_region[region].append(ticket['user']) issues_by_isp[isp].append(ticket['user'])
For each ticket in the tickets list:
- The metadata for the ticket's IP address is fetched using the previously defined get_ip_metadata function.
- The region associated with the IP is extracted and stored in the region variable.
- The ISP associated with the IP is extracted and stored in the isp variable.
- The user's name from the ticket is appended to the list associated with the region in issues_by_region.
- Similarly, the user's name is appended to the list associated with the ISP in issues_by_isp.
5. Printing the Results:
print("Issues by Region:", issues_by_region)
print("Issues by ISP:", issues_by_isp)
The categorized tickets (by region and by ISP) are printed to the console.
In summary, the code takes a list of tickets with user names and IP addresses, fetches metadata for each IP address using the IP stack API, and then categorizes the tickets based on region and ISP. Finally, the categorized lists are printed to provide insights into the distribution of reported issues.
Step 3: Tailored Solutions
With a clearer picture of where and possibly why users are experiencing problems, your support team can prioritize their efforts.
- Region-specific issues: These could be linked to localized server outages or regional network problems. The support team could provide alternative servers or routes for affected users.
- ISP-specific issues: Sometimes, certain ISPs might have issues connecting to your servers. Having this information allows you to liaise directly with the ISP or guide your users on alternative connectivity solutions.
Conclusion
IP stack isn't merely a tool—it's akin to a looking glass, revealing the intricate digital lives of users from all corners of the globe. With the world becoming more interconnected every day, understanding users' geographic and digital contexts has never been more crucial. Businesses equipped with such insights are empowered to provide not just generic support, but highly personalized and location-aware solutions. This is the very essence of modern customer service, transcending borders and addressing individual needs.
However, all these potentials hinge on one critical factor: the accuracy and reliability of the data you work with. IP stack, with its commitment to quality and real-time data provision, stands out in this regard. In today's fast-paced digital world, delays or inaccuracies can lead to missed opportunities and, even worse, disappointed customers. Swift, precise resolutions are not just desired in such an environment; they're a baseline expectation.
If you're a business aiming to elevate your customer support, differentiate your services, or simply gain deeper insights into your user base, then IP stack is an indispensable asset. By providing you with accurate geolocation data and a host of other metadata, it's not just about knowing where your users are—it's about understanding their context, optimizing their experiences, and building lasting relationships.
So, why wait? Dive into the vast ocean of opportunities with IP stack and witness firsthand the transformative power of accurate geolocation data in shaping the future of customer engagement.
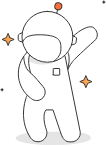